
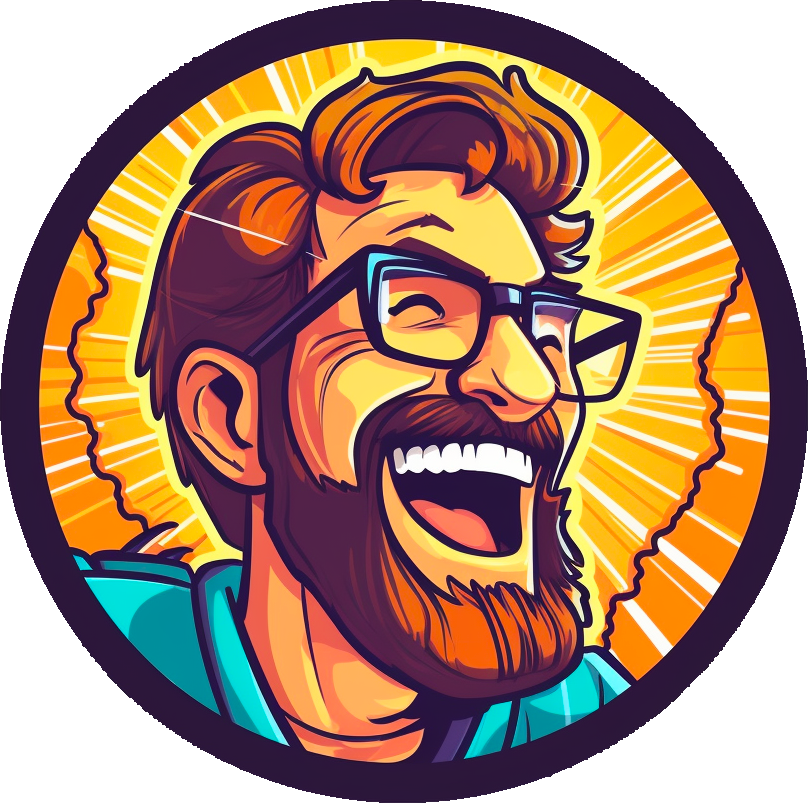
6·
24 hours agoC++ templates my beloved
#pragma pack(1)
template <size_t i> struct increment;
template <> struct increment<0> {
char _plusone;
constexpr static size_t value = 1;
};
template <size_t i> struct increment<i> {
increment<i - 1> _base;
char _plusone; // this increments i
constexpr static size_t value = sizeof(increment<i - 1>);
};
template <size_t i>
using increment_v<i> = increment<i>::value;
This could even be made generic to any integer type with a little more effort
Bonus : to use it without knowing i at compile-time :
template <size_t current_value = 0> size_t& inc(size_t& i) { if (i == current_value) { i = increment<current_value>::value; return i; } else { if constexpr (current_value != std::numeric_limits<size_t>::max()) { return inc<increment<current_value>::value>(i); } } } int main() { int i; std::cin >> i; inc(i); // this increments i std::cout << i << std::endl; return 0; }
Hope you’re not in a hurry